|

The Developer's Resource & Community Site
|
EJB Components
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: EJB Object, Home Object, Remote Object
Pre-required Reading: Enterprise JavaBeans
Let us take an in-depth look at some of the major components of the EJB architecture shown in Figure below and understand their runtime behavior.
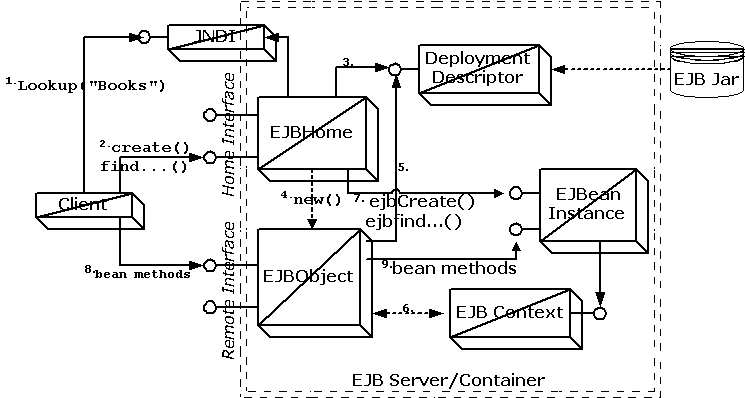 Major components of the EJB Architecture
The Home interface and the Home object
When an EJB client needs to use the services of an enterprise Bean, it creates the EJB through its Home interface. The client specifically uses one of the multiple create() methods that the Home interface defines. The implementation of the Home interface is done through an object called the Home object. An instance of this Home object is created within the server and is made available to the clients as a factory for creating the enterprise Bean.
Finding the Home Object
The EJB client locates the Home object through JNDI since a reference to the home object is placed in the naming service. The location of the namespace and the JNDI context factory class name are provided to the client initially. In addition to providing the location and class name, the client should also have some knowledge of how to locate the Home object within the naming tree.
When an EJB deployer deploys an EJB onto an EJB server, she specifies a particular location in the naming tree such as "com/gopalan/Account". Then, the EJB client must be given this fully qualified pathname to locate and obtain a reference to the "Account" Home object.
// get the JNDI naming context
Context initialCtx = new InitialContext ();
// use the context to lookup the EJB Home interface
AccountHome home =(AccountHome)initialCtx.lookup ("com/gopalan/Account");
// use the Home Interface to create a Session Bean object
Account account = home.create (1234, "Athul", 1000671.54d);
Definition of the Home Interface
The EJBHome object is an implementation of the javax.ejb.EJBHome interface. It has the needed create() and find() methods, and each of these is matched with an ejbCreate() or ejbFind() method of the same signature in the actual Enterprise Bean implementation that is being created. There are also ejbRemove() methods that use the Handle of the EJBean or its Primary Key. There is also a method to obtain the EJBMetaData of the EJBean. This interface is defined in the specification as follows:
public interface javax.ejb.EJBHome extends Remote {
public abstract void remove (Handle handle)
throws RemoteException, RemoveException;
public abstract void remove (Object primaryKey)
throws RemoteException,RemoveException;
public abstract EJBMetaData getEJBMetaData ()
throws RemoteException;
}
The EJB developer has to define ejbCreate() methods in his enterprise beans. He is also required to define corresponding create() methods which match the signatures in the EJB’s home interface. If the developer is coding an Entity Bean, she may have to define finder methods in the home interface which will allow clients to locate existing entity beans based on their identity.
A typical Home interface definition for an EJB may look something like this:
import javax.ejb.*;
import java.rmi.*;
public interface AccountHome extends EJBHome {
Account create(int accountNo, String customer)
throws CreateException, RemoteException;
Account create(int accountNo, String customer, double startingBalance)
throws CreateException, RemoteException;
Account findByPrimaryKey(AccountPK accountNo)
throws FinderException, RemoteException;
}
The Remote Interface
The EJB developer must create a remote interface, which describes the business methods of the Bean that the EJB client would be able to invoke. The EJBObject will have the implementation code generated by the container tools for this interface.
The method names and the signatures listed in the remote interface must exactly match the method names and signatures of the business methods defined by the enterprise Bean. This differs from the Home interface, whose method signatures matched, but the names were different.
A typical remote interface definition for an EJB may look like this.
import javax.ejb.*;
import java.rmi.*;
public interface Account extends EJBObject {
void credit (double amount) throws RemoteException;
void debit (double amount) throws RemoteException;
double getBalance () throws RemoteException;
}
The EJBObject
The EJBObject is a network-visible object with a stub and skeleton that acts as a proxy for the enterprise Bean. The Bean’s remote interface extends the EJBObject interface, making the EJBObject class specific to the Bean class. For each enterprise Bean, there will be a custom EJBObject class.
Types of EJBs: Entity and Session Beans
Enterprise Beans are building blocks that can be used alone or with other enterprise beans to build complete, robust, thin-client multi-tiered applications. An EJB is a body of code with fields and methods to implement modules of business logic. They can either be transient or persistent. There can be two types of enterprise Beans:
- Entity Beans – Beans that are generally used to model a business entity
- Session Beans – general purpose server-side beans
Figure below illustrates a high-level view of an EJB environment with session and entity enterprise beans.
 A typical EJB environment with entity and session beans
Before we begin our discussion of entity and Session Beans, it is important to understand the concept of Passivation and Activation. Passivation is the process by which the state of a Bean is saved to persistent storage and then is swapped out. Activation is the process by which the state of a Bean is restored by swapping it in from persistent storage.
What do you think of this article?
Have your say about the article. You can make your point about the article by mailing [email protected] (If you haven't allready joined, you can join by going to https://www.onelist.com/community/dev-java).
You can also write a review. We will publish the best ones here on this article. Send your review to [email protected]. Please include the title of the article you are reviewing.
Further Reading
The Enterprise JavaBeans Series:
Enterprise Java Beans By Gopalan Suresh Raj.
In this introduction to Enterprise Java Beans, Gopalan covers the bases then goes on to demonstrate how to build server side business object components. This article is the introduction to Gopalans series of Enterprise JavaBeans articles. (This series of articles is courtesy of Gopalan Suresh Raj)
Author: Gopalan Suresh Raj
Date Submitted: January 6th 2000
Level of Difficulty: Advanced
Subjects Covered: Enterprise JavaBeans, EJB Server, EJB Architecture, Java Naming and Directory Interface, Java Transaction Service.
Pre-required Reading: None
Enterprise Java Beans Series - Components at the Server By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Server side components, CORBA
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - EJB Model By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Enterprise JavaBeans, EJB Server, EJB Containers, EJB Clients.
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - EJB Naming Services and JNDI By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Naming Services, Java Naming Directory Interface
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - EJB Transactions and JTS By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Java Transaction Service, Two-phase commits
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - EJB Lifecycle By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: EJB server provider, EJB container provider, EJB developer, EJB deployer, Application developer.
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - EJB Servers By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Server Infrastructure
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - EJB Containers By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: EJB Containers, EJB Servers
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - EJB Session Beans By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Stateful Session Beans, Stateless Session Beans
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - EJB Entity Beans By Gopalan Suresh Raj.
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Container-Managed Persistence, Bean-Managed Persistence
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - Writing an Entity Bean By Gopalan Suresh Raj.
Part 1 of a four part series: A four tier bank account example
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Developing Entity Beans, Home & Remote Interfaces, Data Sources.
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - Writing a Session Bean By Gopalan Suresh Raj.
Part 2 of a four part series: A four tier bank account example
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Developing Session Beans, Home & Remote Interfaces, Deployment Descriptors.
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - Writing an EJB Client By Gopalan Suresh Raj.
Part 3 of a four part series: A four tier bank account example
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: EJB Clients.
Pre-required Reading: Enterprise JavaBeans
Enterprise Java Beans Series - Writing an EJB Servlet Client By Gopalan Suresh Raj.
Part 4 of a four part series: A four tier bank account example
Author: Gopalan Suresh Raj
Date Submitted: January 11th 2000
Level of Difficulty: Advanced
Subjects Covered: Servlet Clients.
Pre-required Reading: Enterprise JavaBeans
Author: Gopalan Suresh Raj
Gopalan has his own site at Author Central (visit him. He also maintains his own site at https://www.execpc.com/~gopalan/) - Contribute to iDevResource.com and you can have one too!
© Copyright 1997-2000 Gopalan Suresh Raj. Reproduced with Permission
|